Setting Up Puppeteer
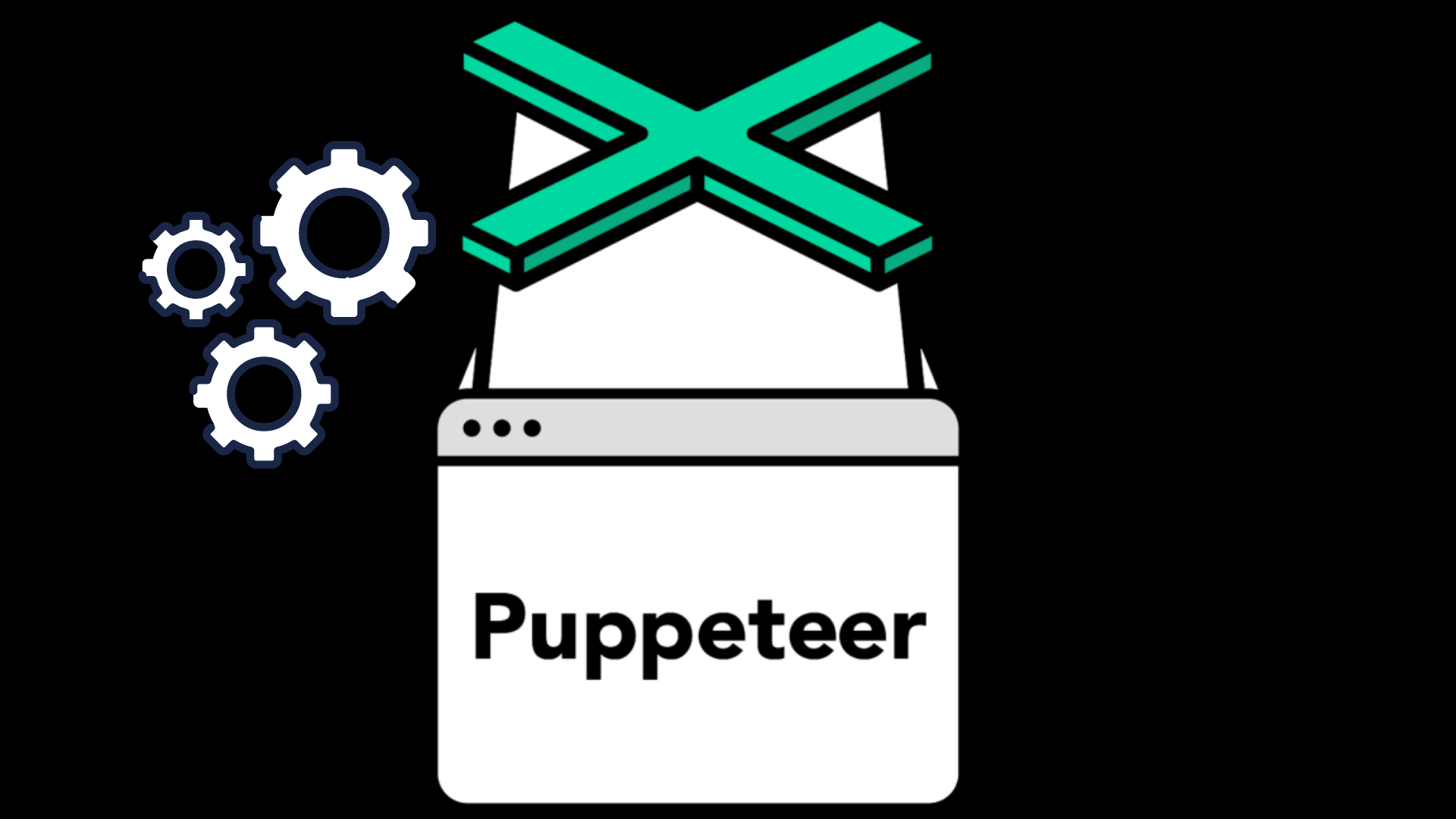
Let’s start by creating a new directory and navigating to it. Assuming you already have Node.js available in your local environment, installing Puppeteer is achieved with just one instruction:
$ npm i puppeteer
Puppeteer come bundled with their respective browsers, so we now have all we need to run our first script. Let’s create a script to navigate to our test website:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch()
const page = await browser.newPage()
await page.goto('https://examplesite.com');
await browser.close()
})()
Run this example as follows:
$ node script.js
Nothing much has happened, right? Remember: by default, Puppeteer will run in headless mode! That means we won’t see anything of what is happening in the browser when our script runs.
Puppeteer creates its own browser user profile, which it cleans up on every run. In other words: all runs will be sandboxed and not interfere with one another, as state is always fully reset at the end of a session.
When you are first writing and debugging your scripts, it is a good idea to disable headless mode, so you can have a look at what your script is doing:
const browser = await puppeteer.launch({ headless: false });